As the demand for high-performance web applications continues to grow, FastAPI has emerged as a popular choice among developers for building robust and efficient APIs. Whether you're a seasoned developer or just starting your journey with FastAPI, preparing for technical interviews in this field is crucial. This comprehensive guide will walk you through essential FastAPI interview questions, best practices, and tips to help you succeed in your next interview.
Introduction to FastAPI Interview Questions
FastAPI has gained significant traction in the Python web development community due to its speed, simplicity, and modern features. As more companies adopt this framework, the demand for skilled FastAPI developers has increased, making it essential for job seekers to be well-prepared for interviews in this domain.
Overview of FastAPI
FastAPI is a modern, fast (high-performance) web framework for building APIs with Python 3.6+ based on standard Python type hints. It's designed to be easy to use, fast to code, ready for production, and suitable for building robust and scalable applications.
Importance of Preparing for FastAPI Interviews
Preparing for FastAPI interviews is crucial for several reasons:
- Demonstrates expertise: Thorough preparation showcases your in-depth knowledge of the framework.
- Builds confidence: Being well-prepared helps you feel more confident during the interview.
- Highlights problem-solving skills: Many interview questions focus on real-world scenarios, allowing you to demonstrate your problem-solving abilities.
- Shows commitment: Investing time in preparation indicates your dedication to the role and the technology.
Common FastAPI Interview Questions
Let's dive into some common FastAPI interview questions you might encounter during your interview process.
General FastAPI Questions
What is FastAPI?
Sample Question: "Can you explain what FastAPI is and its primary purpose?"
Expert Answer: FastAPI is a modern, fast (high-performance) web framework for building APIs with Python 3.6+ based on standard Python type hints. Its primary purpose is to provide a simple and efficient way to create APIs while offering automatic API documentation, request validation, and serialization. FastAPI is built on top of Starlette for the web parts and Pydantic for the data parts, making it both powerful and easy to use.
What are the key features of FastAPI?
Sample Question: "What are some of the standout features that make FastAPI popular among developers?"
Expert Answer: FastAPI offers several key features that contribute to its popularity:
- Fast performance: It's one of the fastest Python frameworks available.
- Easy to use: Intuitive design makes it simple to build APIs quickly.
- Automatic API documentation: Generates interactive API docs (Swagger UI and ReDoc) automatically.
- Data validation: Uses Pydantic for request and response validation.
- Asynchronous support: Built on Starlette, it supports asynchronous programming.
- Type hints: Leverages Python's type hinting for better code quality and IDE support.
- Security features: Includes built-in security utilities like OAuth2 with JWT tokens.
- Dependency injection system: Simplifies the management of dependencies in your application.
How does FastAPI compare to other frameworks like Flask and Django?
Sample Question: "Can you compare FastAPI with Flask and Django in terms of performance and use cases?"
Expert Answer: FastAPI, Flask, and Django are all popular Python web frameworks, but they have different strengths and use cases:
- FastAPI:
- Fastest among the three for API development
- Focuses on building APIs with automatic documentation
- Great for microservices and high-performance requirements
- Newer, with a growing ecosystem
- Flask:
- Lightweight and flexible
- Good for small to medium-sized applications
- Requires more manual configuration
- Large ecosystem of extensions
- Django:
- Full-featured framework with built-in admin interface
- Best for large, complex applications with many features
- Includes ORM and many built-in tools
- Steeper learning curve but powerful for full-stack development
FastAPI excels in API development and performance, Flask is great for flexibility, and Django is ideal for full-featured web applications.
Technical FastAPI Questions
Explain how FastAPI handles request validation.
Sample Question: "How does FastAPI ensure that incoming requests meet the expected format and data types?"
Expert Answer: FastAPI uses Pydantic models for request validation. When you define your API endpoints, you can specify the expected request body, query parameters, and path parameters using Pydantic models or Python type annotations. FastAPI automatically validates incoming requests against these definitions, ensuring that:
- Required fields are present
- Data types match the specified types
- Additional validation rules (like min/max values) are enforced
If a request doesn't meet these criteria, FastAPI automatically returns a detailed error response, saving developers from writing extensive validation code.
What are dependencies in FastAPI?
Sample Question: "Can you explain the concept of dependencies in FastAPI and provide an example of how they're used?"
Expert Answer: Dependencies in FastAPI are a way to declare shared logic, database connections, or any reusable code that can be injected into path operation functions. They help in:
- Code reuse and organization
- Automatic request validation
- Implementing security and authentication
Here's a simple example:
from fastapi import Depends, FastAPI
app = FastAPI()
def get_query_param(q: str = None):
return q
@app.get("/items/")
async def read_items(query: str = Depends(get_query_param)):
return {"query": query}
In this example, get_query_param
is a dependency that extracts and optionally validates a query parameter. It's injected into the read_items
function using Depends()
.
How does FastAPI support asynchronous programming?
Sample Question: "Explain how FastAPI leverages asynchronous programming and its benefits."
Expert Answer: FastAPI fully supports asynchronous programming through its integration with Starlette and the async
/await
syntax in Python. Here's how it works:
- Async route handlers: You can define route handlers as async functions.
- Async dependencies: Dependencies can also be async functions.
- Concurrent execution: FastAPI can handle multiple requests concurrently without blocking.
Benefits include:
- Improved performance for I/O-bound operations
- Better scalability, especially for applications with many concurrent users
- Efficient use of system resources
Example:
@app.get("/items/{item_id}")
async def read_item(item_id: int):
item = await database.fetch_one(f"SELECT * FROM items WHERE id = {item_id}")
return item
This async route handler can efficiently handle database queries without blocking other requests.
Can you explain the use of path and query parameters in FastAPI?
Sample Question: "How do you define and use path and query parameters in FastAPI routes?"
Expert Answer: FastAPI makes it easy to work with both path and query parameters:
- Path Parameters:
- Defined in the route using curly braces
{}
- Extracted from the URL pathExample:
@app.get("/items/{item_id}")
def read_item(item_id: int):
return {"item_id": item_id}
- Query Parameters:
- Defined as function parameters
- Extracted from the URL query stringExample:
@app.get("/items/")
def list_items(skip: int = 0, limit: int = 10):
return {"skip": skip, "limit": limit}
FastAPI automatically validates and converts these parameters to the specified types, raising appropriate errors if the input is invalid.
How do you implement authentication and authorization in FastAPI?
Sample Question: "Describe a common approach to implementing JWT-based authentication in a FastAPI application."
Expert Answer: Implementing JWT-based authentication in FastAPI typically involves these steps:
- Use FastAPI's security utilities:
from fastapi.security import OAuth2PasswordBearer
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
- Create a route for token generation:
@app.post("/token")
async def login(form_data: OAuth2PasswordRequestForm = Depends()):
# Validate user credentials
# Generate and return JWT token
- Use dependencies to protect routes:
@app.get("/users/me")
async def read_users_me(current_user: User = Depends(get_current_user)):
return current_user
- Implement the
get_current_user
dependency:
async def get_current_user(token: str = Depends(oauth2_scheme)):
# Decode and validate JWT token
# Return user or raise HTTPException
This approach ensures that protected routes can only be accessed with a valid JWT token, providing a secure authentication mechanism.
Advanced FastAPI Interview Questions
Performance and Scalability
What makes FastAPI a high-performance framework?
Sample Question: "Can you explain the key factors that contribute to FastAPI's high performance?"
Expert Answer: FastAPI's high performance is attributed to several factors:
- Starlette: Built on top of Starlette, a lightweight ASGI framework.
- Pydantic: Uses Pydantic for data validation, which is implemented in Cython for speed.
- Asynchronous support: Leverages Python's async capabilities for non-blocking I/O operations.
- Just-in-time compilation: Utilizes Pydantic's JIT compiler for optimized data processing.
- Minimal overhead: Designed to add minimal overhead to your application logic.
- Type hints: Uses Python's type hints for both improved performance and developer experience.
These features combined allow FastAPI to handle a high number of requests with low latency, making it suitable for high-performance applications.
How can you optimize FastAPI applications for better performance?
Sample Question: "What strategies would you employ to improve the performance of a FastAPI application?"
Expert Answer: To optimize a FastAPI application for better performance, consider the following strategies:
- Use asynchronous programming: Leverage
async
/await
for I/O-bound operations. - Implement caching: Use Redis or in-memory caching for frequently accessed data.
- Database optimization: Use efficient queries and indexing in your database.
- Connection pooling: Implement connection pooling for database connections.
- Use background tasks: Offload time-consuming tasks to background workers.
- Optimize dependencies: Minimize the use of heavy dependencies in frequently called paths.
- Use Uvicorn with Gunicorn: Deploy with Uvicorn workers managed by Gunicorn for better concurrency.
- Content compression: Enable Gzip compression for responses.
- Profiling and monitoring: Regularly profile your application to identify bottlenecks.
Implementing these strategies can significantly improve the performance and scalability of your FastAPI application.
Testing and Debugging
How do you test FastAPI applications?
Sample Question: "Describe your approach to testing FastAPI applications, including the tools and methodologies you use."
Expert Answer: Testing FastAPI applications typically involves:
- Unit Testing:
- Use
pytest
for writing and running tests - Test individual functions and classes in isolation
- Integration Testing:
- Use FastAPI's
TestClient
for testing API endpoints - Test the interaction between different components
- Mocking:
- Use
unittest.mock
orpytest-mock
to mock external dependencies
- Parameterized Testing:
- Use
pytest.mark.parametrize
for testing multiple scenarios
Example of a simple test using TestClient
:
from fastapi.testclient import TestClient
from main import app
client = TestClient(app)
def test_read_main():
response = client.get("/")
assert response.status_code == 200
assert response.json() == {"message": "Hello World"}
This approach ensures comprehensive testing of your FastAPI application, covering both individual components and the API as a whole.
What tools do you use for debugging FastAPI applications?
Sample Question: "What debugging tools and techniques do you find most effective when working with FastAPI?"
Expert Answer: For debugging FastAPI applications, I typically use a combination of tools and techniques:
- Python Debugger (pdb): For step-by-step debugging of Python code.
- IDE Debuggers: Such as PyCharm or VS Code debuggers for a more visual debugging experience.
- Logging: Implement detailed logging using Python's
logging
module or a third-party logger likeloguru
. - FastAPI's built-in error handling: Utilize FastAPI's automatic error responses for debugging during development.
- API Documentation: Use the auto-generated Swagger UI for testing and debugging endpoints.
- Network Inspection Tools: Like Postman or cURL for inspecting API requests and responses.
- Performance Profilers: Tools like
cProfile
orpy-spy
for identifying performance bottlenecks.
These tools, combined with FastAPI's clear error messages and stack traces, make debugging a more straightforward process.
FastAPI and Databases
How does FastAPI integrate with databases?
Sample Question: "Explain how you would typically integrate a database with a FastAPI application."
Expert Answer: FastAPI can integrate with databases in several ways:
- ORM Integration:
- Use ORMs like SQLAlchemy or Tortoise-ORM
- Define database models and use them in your FastAPI routes
- Async Database Libraries:
- Utilize async database libraries like
databases
orasyncpg
for PostgreSQL - Leverage FastAPI's async support for non-blocking database operations
- Connection Management:
- Use dependency injection to manage database connections
- Implement connection pooling for efficient resource usage
Example using SQLAlchemy:
from fastapi import FastAPI, Depends
from sqlalchemy.orm import Session
from . import crud, models, schemas
from .database import SessionLocal, engine
app = FastAPI()
# Dependency
def get_db():
db = SessionLocal()
try:
yield db
finally:
db.close()
@app.post("/users/", response_model=schemas.User)
def create_user(user: schemas.UserCreate, db: Session = Depends(get_db)):
return crud.create_user(db=db, user=user)
This approach allows for efficient and organized database interactions within your FastAPI application.
What ORM (Object-Relational Mapping) libraries can be used with FastAPI?
Sample Question: "What are some popular ORM choices for FastAPI, and what are their pros and cons?"
Expert Answer: Several ORM libraries can be used effectively with FastAPI:
- SQLAlchemy:
- Pros: Mature, feature-rich, supports many databases
- Cons: Can be complex for simple use cases, async support is relatively new
- Tortoise-ORM:
- Pros: Async-first design, easy to use with FastAPI
- Cons: Less mature than SQLAlchemy, fewer features for complex scenarios
- Peewee:
- Pros: Lightweight, simple to use
- Cons: Limited async support, not as feature-rich as SQLAlchemy
- Pony ORM:
- Pros: Intuitive query syntax, good for small to medium projects
- Cons: Limited async support, smaller community compared to SQLAlchemy
The choice of ORM depends on your project requirements, such as the need for async operations, database complexity, and performance considerations.
Ace Your FastAPI Interview with TalenCat CV Maker
Preparing for a FastAPI interview can be challenging, but with the right tools, you can significantly boost your chances of success. TalenCat CV Maker, an AI-powered online resume builder, offers a unique feature to help you navigate potential FastAPI interview questions based on your resume content.
Here's how to use TalenCat CV Maker to prepare for your FastAPI interview:
Step 1: Sign up or log in to TalenCat CV Maker. Create a new resume tailored for your FastAPI developer role or upload your existing one.
Step 2: Navigate to the "AI Assistant" section and select "Interview Assistant" from the left-side menu. This powerful tool will analyze your resume content specifically for FastAPI-related information.

Step 3: Click "Analyze Now" to generate potential FastAPI interview questions based on your resume content. The AI will focus on your FastAPI experience, projects, and skills to create relevant questions.

Step 4: Review the generated questions and prepare your answers. These questions will likely cover FastAPI-specific topics such as:
- Asynchronous programming in FastAPI
- Dependency injection
- API documentation with Swagger UI
- Database integration with FastAPI
- Authentication and authorization in FastAPI applications
Step 5: Use the TalenCat CV Maker's AI-powered editor to refine your resume further, highlighting your FastAPI expertise and projects.
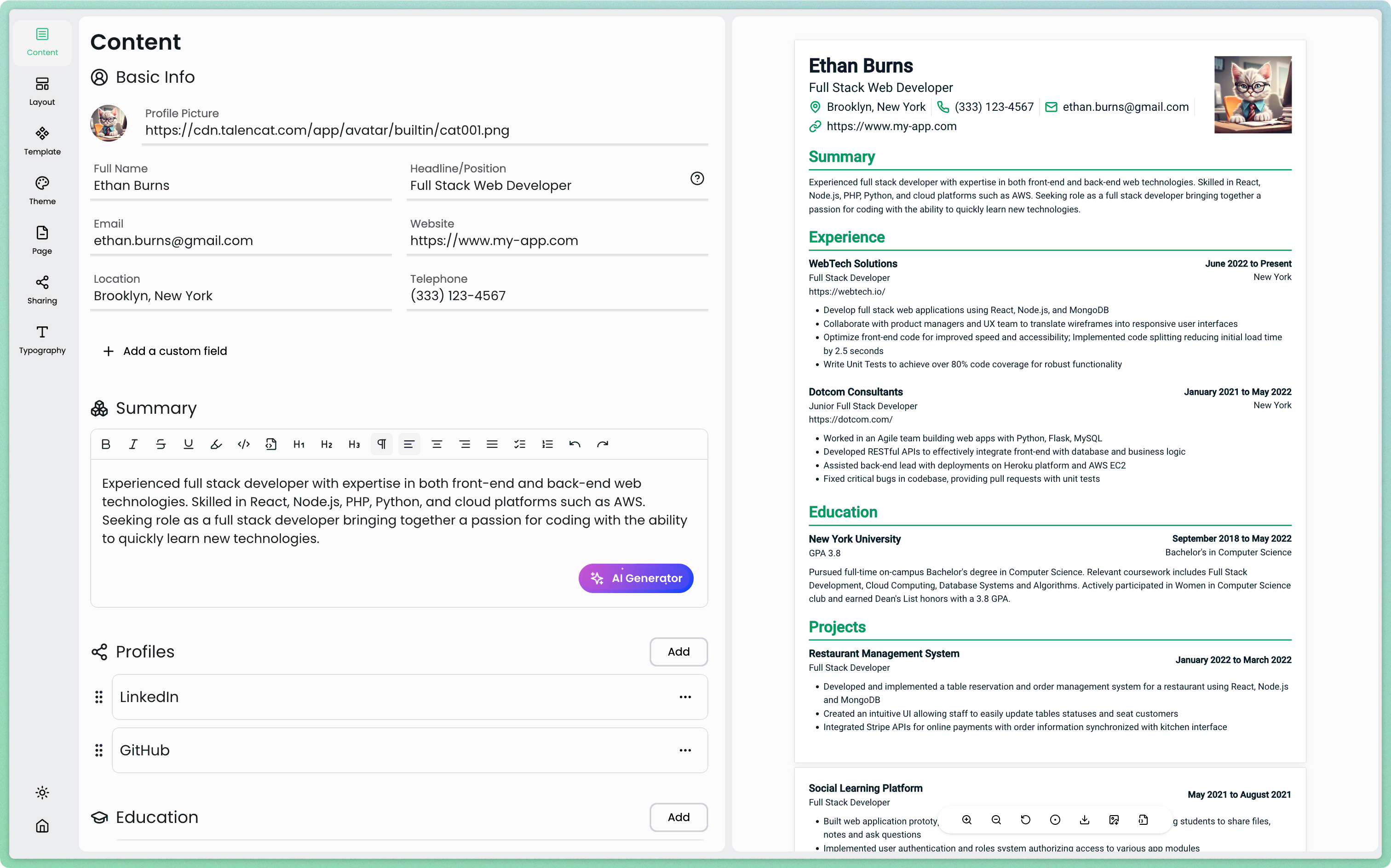
By leveraging TalenCat CV Maker's Interview Assistant, you'll be well-prepared to tackle even the most challenging FastAPI interview questions. This tool not only helps you anticipate potential questions but also ensures that your resume effectively showcases your FastAPI skills.
Remember, the key to a successful interview is thorough preparation. With TalenCat CV Maker, you're not just creating a resume; you're strategically positioning yourself for success in your FastAPI developer interview.
Best Practices for FastAPI Development
Coding Standards and Organization
When working with FastAPI, it's important to follow Python's PEP 8 style guide and organize your code in a logical structure. Here are some best practices:
- Use clear and descriptive names for variables, functions, and classes.
- Organize your project into modules (e.g.,
models.py
,schemas.py
,crud.py
). - Implement dependency injection for better code organization and testability.
- Use type hints consistently to improve code readability and catch errors early.
- Keep your route handlers focused and delegate complex logic to separate functions or classes.
Documentation and API Standards
Documentation is crucial for maintaining and scaling FastAPI applications. Key practices include:
- Write clear docstrings for all functions and classes
- Use OpenAPI (Swagger) documentation effectively
- Include example requests and responses
- Document error scenarios and handling
- Maintain consistent API naming conventions
- Include version control in your API endpoints
- Document authentication and authorization requirements
Tips for Success in FastAPI Interviews
Researching the Company and Role
Before your interview:
- Study the company's tech stack and how they use FastAPI
- Review their public APIs if available
- Understand their business domain and challenges
- Research their development culture and practices
- Prepare questions about their FastAPI implementation
Practicing Common Interview Questions
Focus on:
- Core FastAPI concepts and features
- Performance optimization techniques
- Security implementation
- Database integration
- Testing methodologies
- Real-world problem-solving scenarios
- System design using FastAPI
Engaging in Mock Interviews
Prepare through:
- Practice with peers or mentors
- Record yourself answering questions
- Time your responses
- Get feedback on your explanations
- Practice coding challenges specific to FastAPI
- Simulate technical discussions
Resources for Further Learning
Recommended Books and Online Courses
- Books:
- "FastAPI: Modern Python Web Development"
- "Building Data Science Applications with FastAPI"
- "Python API Development Fundamentals"
- Online Courses:
- Udemy's FastAPI Complete Course
- TestDriven.io FastAPI Tutorials
- Real Python's FastAPI Learning Path
Useful FastAPI Documentation and Tutorials
- Official Resources:
- FastAPI Official Documentation
- FastAPI GitHub Repository
- Tiangolo's FastAPI Tutorial Series
- Community Resources:
- FastAPI Forum
- Stack Overflow FastAPI Tags
- FastAPI Discord Community
Conclusion
Summary of Key Takeaways
- FastAPI is a modern, high-performance framework for building APIs
- Success requires understanding both basic and advanced concepts
- Practice and preparation are essential for interviews
- Keep up with best practices and community standards
- Continuous learning is key in the FastAPI ecosystem
Encouragement for Candidates Preparing for FastAPI Interviews
- Focus on hands-on practice with real projects
- Build a portfolio of FastAPI applications
- Engage with the FastAPI community
- Stay updated with the latest features and updates
- Remember that understanding core concepts is more important than memorizing syntax