As the demand for efficient and performant web applications continues to grow, Next.js has emerged as a powerful framework for building modern web applications. This comprehensive guide will walk you through essential Next.js interview questions, providing you with the knowledge and confidence to excel in your next interview. Whether you're a seasoned developer or just starting with Next.js, this article will cover a wide range of topics to help you prepare for your upcoming interview.
Introduction to Next.js Interview Questions
Next.js has revolutionized the way developers build React applications, offering a robust set of features that enhance performance, SEO, and developer experience. As more companies adopt Next.js for their web projects, the demand for skilled Next.js developers has skyrocketed. This guide will help you navigate through common interview questions and provide expert insights into the framework's core concepts.
Importance of Next.js in Modern Web Development
Next.js has become a cornerstone in modern web development due to its ability to solve common challenges faced by developers. It offers server-side rendering, static site generation, and client-side rendering out of the box, making it an ideal choice for building scalable and performant web applications. Understanding Next.js is crucial for developers looking to stay competitive in the ever-evolving landscape of web development.
Overview of Next.js Framework
Next.js is a React-based framework that enables functionality such as server-side rendering and generating static websites for React-based web applications. It's designed to enhance the developer experience by providing a set of powerful features and optimizations right out of the box. As we delve into the interview questions, you'll gain a deeper understanding of these features and how they contribute to building efficient web applications.
Common Next.js Interview Questions
This section covers the most frequently asked questions in Next.js interviews, ranging from basic concepts to more technical aspects of the framework.
General Questions
What is Next.js?
Sample Question: Can you explain what Next.js is and its primary purpose?
Expert Answer: Next.js is a React framework that enables server-side rendering, static site generation, and other performance optimizations for React applications. It's designed to make it easy to build scalable, production-ready web applications with React. Next.js handles the tooling and configuration needed for React, and provides additional structure, features, and optimizations.
What are the key features of Next.js?
Sample Question: What are some of the standout features that make Next.js popular among developers?
Expert Answer: Next.js offers several key features that make it popular:
- Server-Side Rendering (SSR) for improved performance and SEO
- Static Site Generation (SSG) for fast, pre-rendered pages
- Automatic code splitting for faster page loads
- Built-in CSS support
- API routes for building API endpoints
- Easy deployment with platforms like Vercel
- TypeScript support out of the box
- Image optimization
- Fast Refresh for instant feedback during development
How does Next.js differ from React?
Sample Question: Can you explain the main differences between Next.js and React?
Expert Answer: While Next.js is built on top of React, it provides additional features and optimizations. React is a library for building user interfaces, while Next.js is a full framework that includes React and adds server-side rendering, routing, and build optimizations. Next.js simplifies many aspects of building React applications, such as code splitting and server-side rendering, which would require additional setup and configuration in a plain React application.
Technical Questions
Explain the difference between SSR, SSG, and CSR.
Sample Question: Can you describe the differences between Server-Side Rendering (SSR), Static Site Generation (SSG), and Client-Side Rendering (CSR) in Next.js?
Expert Answer: These are different approaches to rendering web pages:
- SSR (Server-Side Rendering): The server generates the full HTML for each request. This is good for dynamic content and SEO but can be slower for complex pages.
- SSG (Static Site Generation): Pages are generated at build time and can be served as static files. This is fastest for delivery but not suitable for highly dynamic content.
- CSR (Client-Side Rendering): The initial HTML is minimal, and JavaScript renders the content in the browser. This can provide a rich, app-like experience but may impact initial load time and SEO.
Next.js allows you to choose the most appropriate rendering method for each page in your application.
What is getStaticProps and getServerSideProps?
Sample Question: Can you explain the purpose and differences between getStaticProps and getServerSideProps in Next.js?
Expert Answer: Both are Next.js data fetching methods:
- getStaticProps runs at build time in production and allows you to fetch data and render pages as static HTML.
- getServerSideProps runs on every request, allowing you to render pages dynamically on the server.
The main difference is that getStaticProps is for static data that can be known at build time, while getServerSideProps is for data that must be fetched for every request.
How do you handle routing in Next.js?
Sample Question: Describe how routing works in Next.js and how it differs from traditional React routing.
Expert Answer: Next.js uses a file-system based routing. Pages are associated with a route based on their file name within the pages directory. For example, pages/about.js would be accessible at /about. This approach simplifies routing compared to traditional React routing where routes are typically defined manually. Next.js also supports dynamic routes and nested routing, making it flexible for various application structures.
What are dynamic routes in Next.js?
Sample Question: Can you explain what dynamic routes are in Next.js and provide an example of how they're implemented?
Expert Answer: Dynamic routes in Next.js allow you to create pages with paths that depend on external data. For example, you might have a file named [id].js in your pages/posts directory. This would match any route like /posts/1, /posts/2, etc. You can then use the id parameter in your page component to fetch the appropriate data. This feature is particularly useful for creating pages that display content based on URL parameters.
How can you implement API routes in Next.js?
Sample Question: Describe how to create and use API routes in a Next.js application.
Expert Answer: API routes in Next.js allow you to create API endpoints as part of your Next.js application. To create an API route, you add a file to the pages/api directory. For example, pages/api/users.js could handle requests to /api/users. Inside this file, you export a default function that receives the request and response objects. You can then use these to handle the API logic, such as querying a database or processing data. This feature allows you to build full-stack applications entirely within Next.js.
Performance and Optimization Questions
How does Next.js improve performance?
Sample Question: What are some ways Next.js enhances the performance of web applications?
Expert Answer: Next.js improves performance through several mechanisms:
- Automatic code splitting, which reduces the initial load time by only loading necessary JavaScript.
- Server-side rendering, which improves initial page load and SEO.
- Static site generation for fast, pre-rendered pages.
- Image optimization, automatically serving optimized images for different devices.
- Prefetching of links for faster client-side transitions.
- Built-in CSS support for optimized styling.
What are some strategies for optimizing a Next.js application?
Sample Question: Can you discuss some best practices for optimizing the performance of a Next.js application?
Expert Answer: To optimize a Next.js application, consider:
- Using getStaticProps for static data to leverage static generation.
- Implementing proper code splitting and lazy loading of components.
- Optimizing images using Next.js Image component.
- Minimizing external dependencies and removing unused code.
- Leveraging the built-in performance analytics to identify bottlenecks.
- Using caching strategies for API routes and database queries.
- Implementing proper error boundaries to prevent entire app crashes.
Explain the concept of code splitting in Next.js.
Sample Question: How does code splitting work in Next.js, and why is it important?
Expert Answer: Code splitting in Next.js automatically splits your code into smaller chunks, loading only the necessary JavaScript for each page. This means that when a user visits a page, they download only the code required for that specific page, rather than the entire application bundle. This significantly reduces initial load times, especially for large applications. Next.js handles this automatically for page-level code splitting and also provides tools like dynamic imports for component-level code splitting.
Deployment and Hosting Questions
How do you deploy a Next.js application?
Sample Question: Can you walk me through the process of deploying a Next.js application?
Expert Answer: Deploying a Next.js application typically involves these steps:
- Ensure your application is production-ready and all environment variables are set.
- Choose a hosting platform (e.g., Vercel, Netlify, or custom server).
- If using Vercel (created by the Next.js team), you can simply connect your GitHub repository and it will automatically deploy your app.
- For other platforms, you might need to set up build commands (usually
next build
) and specify the output directory. - Configure any necessary server settings, such as Node.js version.
- Deploy your application and verify it's working correctly in the production environment.
What are the best practices for hosting Next.js applications?
Sample Question: What should be considered when choosing a hosting solution for a Next.js application?
Expert Answer: When hosting a Next.js application, consider:
- Support for server-side rendering if your app uses it.
- Ability to run Node.js applications if you're using API routes.
- Automatic HTTPS and SSL certificate management.
- Easy integration with CI/CD pipelines for automated deployments.
- Scalability to handle traffic spikes.
- Support for environment variables and secrets management.
- CDN integration for improved global performance.
- Monitoring and analytics capabilities.
Platforms like Vercel are optimized for Next.js, but other options like Netlify, AWS, or Google Cloud can also be suitable depending on your specific requirements.
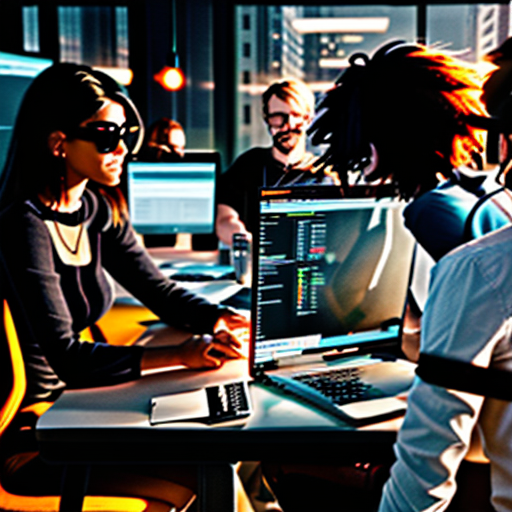
TalenCat CV Maker: Prepare for Your Next.js Interview Questions
When preparing for a Next.js developer interview, it's crucial to anticipate the questions that may arise based on your resume. The TalenCat CV Maker is an exceptional online resume builder that not only helps you create a standout resume but also assists you in navigating potential interview questions tailored to your experience and skills.
Step 1: Log in to TalenCat CV Maker
Begin by logging into the TalenCat CV Maker. You can either create a new resume specifically for your Next.js developer role or upload your existing resume for analysis.
Step 2: Access the AI Assistant
Once logged in, navigate to the left-side menu and select "AI Assistant" followed by "Interview Assistant". This feature will analyze your resume content and provide you with tailored interview questions.

Step 3: Analyze Your Resume
Click on "Analyze Now" to allow TalenCat CV Maker to generate potential interview questions based on the specifics of your resume. This will help you prepare for questions that may focus on your experience with Next.js and related technologies.

With these personalized interview questions, you can confidently prepare for your Next.js developer interview, ensuring that you are ready to showcase your skills and experiences effectively.
Conclusion
Preparing for an interview can be overwhelming, but with the TalenCat CV Maker, you can gain a significant edge. By understanding the potential questions you may face, you can craft thoughtful responses that highlight your qualifications for the Next.js developer position. Take advantage of this powerful AI interview tool to ensure you present yourself in the best possible light during your interview.
Advanced Next.js Interview Questions
State Management in Next.js
How do you manage state in a Next.js application?
Sample Question: What approaches do you use for state management in a Next.js application?
Expert Answer: State management in Next.js can be handled in several ways:
- For local component state, React's useState hook is often sufficient.
- For more complex state, you might use the useReducer hook or Context API for sharing state across components.
- For global state management, libraries like Redux or MobX can be integrated with Next.js.
- Next.js also provides SWR (stale-while-revalidate) for data fetching and caching, which can be used for remote state management.
- For server-side state, you can use getServerSideProps or API routes to fetch and manage data.
The choice depends on the complexity of your application and the specific requirements of your project.
What libraries can be used for state management in Next.js?
Sample Question: Can you name some popular state management libraries used with Next.js and briefly explain their use cases?
Expert Answer: Several state management libraries work well with Next.js:
- Redux: For complex global state management, especially in large applications.
- MobX: Offers a more flexible approach to state management with less boilerplate than Redux.
- Recoil: A Facebook-developed state management library that works well for atomic state management.
- Zustand: A minimalist state management solution that's gaining popularity due to its simplicity.
- Jotai: Another atomic state management library that integrates well with React's concurrent features.
The choice of library depends on factors like the size of your application, the complexity of your state, and your team's familiarity with the library.
Testing and Debugging Questions
How do you test a Next.js application?
Sample Question: What testing strategies and tools do you use for Next.js applications?
Expert Answer: Testing Next.js applications typically involves:
- Unit testing with Jest for individual components and functions.
- Integration testing with React Testing Library to test component interactions.
- End-to-end testing with tools like Cypress or Playwright to test the entire user flow.
- API route testing using supertest or similar libraries.
- Snapshot testing for UI components to detect unexpected changes.
- Mocking external dependencies and API calls for consistent test results.
Next.js provides a testing environment that works out of the box with Jest, making it easier to set up and run tests.
What tools do you recommend for debugging Next.js applications?
Sample Question: What debugging tools and techniques do you find most effective when working with Next.js?
Expert Answer: For debugging Next.js applications, I recommend:
- Chrome DevTools for client-side debugging and performance profiling.
- React Developer Tools for inspecting component hierarchies and props.
- Next.js built-in error reporting for server-side errors.
- Node.js debugging tools for server-side code and API routes.
- Redux DevTools if using Redux for state management.
- Logging libraries like winston or debug for detailed server-side logs.
- Browser extensions like React Developer Tools and Redux DevTools for state and component inspection.
Additionally, using the debugger
statement or console.log
for quick debugging during development can be very helpful.
Behavioral Questions
Experience with Next.js
Describe a project where you used Next.js.
Sample Question: Can you tell me about a significant project you've worked on using Next.js?
Expert Answer: In a recent project, I developed an e-commerce platform using Next.js. We chose Next.js for its server-side rendering capabilities, which significantly improved our site's SEO and initial load times. I implemented dynamic product pages using Next.js's file-based routing system and leveraged getStaticProps for pre-rendering product listings. We also used API routes to handle user authentication and shopping cart functionality. The project showcased Next.js's versatility in building a full-stack application with optimal performance.
What challenges did you face while working with Next.js?
Sample Question: What was the most significant challenge you encountered while working with Next.js, and how did you overcome it?
Expert Answer: One significant challenge I faced was optimizing the performance of a large Next.js application with numerous dynamic routes. The build times were becoming increasingly long, impacting our deployment process. To address this, we implemented incremental static regeneration (ISR) for frequently updated pages, which allowed us to generate static pages at runtime. We also optimized our data fetching strategies and implemented better code splitting. These changes significantly reduced our build times and improved overall application performance.
Problem-Solving Questions
How would you approach a performance issue in a Next.js app?
Sample Question: If you encountered a performance issue in a Next.js application, what steps would you take to diagnose and resolve it?
Expert Answer: To address a performance issue in a Next.js app, I would:
- Use browser developer tools to identify bottlenecks (network, rendering, etc.).
- Analyze the application's bundle size using tools like webpack-bundle-analyzer.
- Check for unnecessary re-renders using React DevTools.
- Optimize images and implement lazy loading where appropriate.
- Review data fetching methods and consider implementing caching strategies.
- Use Next.js's built-in performance analytics to identify slow pages or components.
- Consider implementing code splitting for large components or pages.
- Optimize API routes and database queries if server-side performance is an issue.
The specific approach would depend on the nature of the performance issue identified.
Describe a time when you had to learn a new technology quickly.
Sample Question: Can you share an experience where you had to quickly learn and implement a new technology in a project?
Expert Answer: In a recent project, we needed to implement real-time features in our Next.js application. I had to quickly learn and integrate Socket.IO, which I hadn't used before. I started by reading the official documentation and following tutorials. I then created a small proof-of-concept to understand how it works with Next.js. After gaining confidence, I implemented the real-time features in our main application, continuously refining my approach as I learned more. This experience taught me the importance of rapid learning and adaptability in development.
Conclusion
Recap of Key Points
As we conclude this comprehensive guide to Next.js interview questions, let's recap some key points:
- Advanced state management is crucial for complex Next.js applications
- Testing and debugging require a combination of tools and methodologies
- Real-world experience and problem-solving skills are highly valued
- Understanding performance optimization is essential
- Continuous learning and adaptability are key traits for Next.js developers
Final Tips for Preparing for Next.js Interviews
To excel in your Next.js interview:
- Practice coding exercises focused on Next.js features
- Stay updated with the latest Next.js releases and features
- Build personal projects to gain hands-on experience
- Review common debugging scenarios and solutions
- Prepare concrete examples from your past experiences
- Study both technical and behavioral aspects of interviews
- Focus on understanding core concepts rather than memorizing answers
- Be ready to discuss real-world applications and challenges